C++
Description
The C++ SDK utilizes three open-source libraries for easy integration:
-
HTTP/HTTPS library https://github.com/yhirose/cpp-httplib Used for making HTTP requests.
-
JSON for Modern C++ https://github.com/nlohmann/json Used for parsing JSON strings.
-
PNG encoder and decoder https://github.com/lvandeve/lodepng Used for storing image data.
Interface Definition
struct EpicEyeInfo {
std::string SN; // Serial number
std::string IP; // Camera IP address
std::string model; // Camera model
std::string alias; // Camera alias, which can be changed via Web UI
uint32_t width = 0; // Image width
uint32_t height = 0; // Image height
};
Function Name | Description | Parameters and Return Value |
---|---|---|
|
Retrieve the SDK version |
Return Value: A std::string containing the SDK version number |
|
Get camera information |
Parameters: ip: The IP address of the camera info: An EpicEyeInfo object to receive detailed camera information, including width and height Return Value: A bool indicating whether the request was successful |
|
Trigger capturing a frame |
Parameters: ip: The IP address of the camera frameID: A std::string to receive the frame ID of the captured image pointCloud: A bool indicating whether to request point cloud data Return Value: A bool indicating whether the request was successful |
|
Retrieve a 2D image by frame ID |
Parameters: ip: The IP address of the camera frameID: The frame ID of the data to be retrieved imageBuffer: A void pointer to store the returned image data pixelByteSize: An int to receive the size of the image data in bytes Return Value: A bool indicating whether the request was successful |
|
Retrieve point cloud data by frame ID, with memory allocated and released by the SDK user |
Parameters: ip: The IP address of the camera frameID: The frame ID of the data to be retrieved, obtained via triggerFrame pointCloudBuffer: A float pointer to store the point cloud data, with memory of size width * height * sizeof(float) * 3 managed by the SDK user Return Value: A bool indicating whether the request was successful |
|
Retrieve depth map by frame ID, with memory allocated and released by the SDK user |
Parameters: ip: The IP address of the camera frameID: The frame ID of the data to be retrieved, obtained via triggerFrame depth: A float pointer to store the depth map data, with memory of size width * height * sizeof(float) managed by the SDK user Return Value: A bool indicating whether the request was successful |
|
Retrieve camera configuration by frame ID. If frameID is an empty string "", the latest camera configuration will be returned |
Parameters: ip: The IP address of the camera configJson: An nlohmann::json object to receive the camera configuration data frameID: The frame ID of the data to be retrieved, obtained via triggerFrame Return Value: A bool indicating whether the request was successful |
|
Update camera configuration |
Parameters: ip: The IP address of the camera configJson: An nlohmann::json object containing the configuration data to be set Return Value: A bool indicating whether the request was successful |
|
Retrieve the camera intrinsic matrix |
Parameters: ip: The IP address of the camera cameraMatrix: A std::vector<float> storing the camera matrix in row-major order, compatible with OpenCV Return Value: A bool indicating whether the request was successful |
|
Retrieve camera distortion coefficients |
Parameters: ip: The IP address of the camera distortion: A std::vector<float> containing distortion coefficients, compatible with OpenCV Return Value: A bool indicating whether the request was successful |
|
Automatically search for cameras |
Parameters: cameraList: A std::vector<EpicEyeInfo> to store the list of found cameras Return Value: A bool indicating whether cameras were successfully discovered |
Linux Operation
-
Dependencies: gcc, g++, cmake, make
For Debian-based Linux systems, install with:
sudo apt install gcc g++ cmake make
-
After the environment is set up:
mkdir build && cd build cmake .. && make -j12
The compiled library and
samples
binary files are stored in theoutput
folder:output/ ├── bin │ ├── CameraConfig │ ├── CameraInfo │ ├── CameraParameters │ ├── EpicEyeCapture │ └── SearchCamera └── lib └── libEpicEyeSDK.a
OpenCV Example
-
Install OpenCV environment:
sudo apt install libopencv-dev
-
Alternatively, download the precompiled package: Download OpenCV Precompiled Package
sudo dpkg -i opencv-qytech_4.5.3-5+focal_amd64.deb
Windows Operation
If using CMake and Clang toolchain on Windows, refer to the Linux process.
If using Visual Studio, directly open the cpp
folder via VsCode, as the project has no external dependencies.
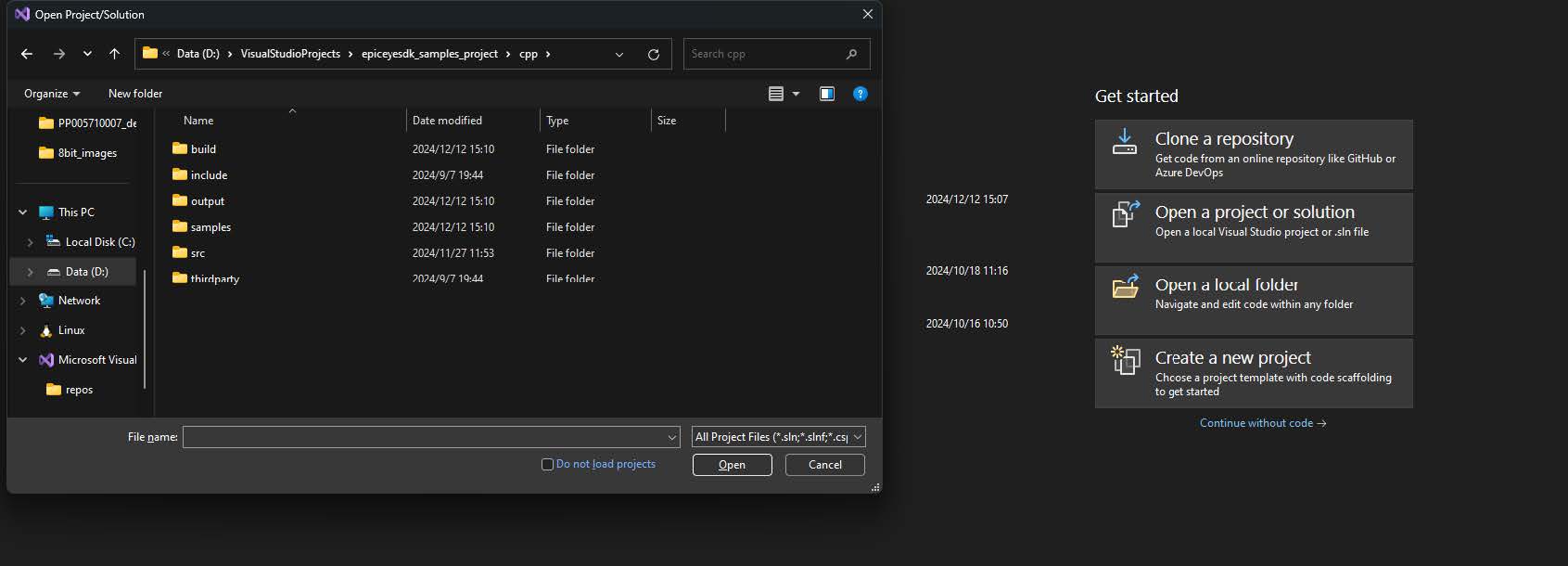
Run all builds:
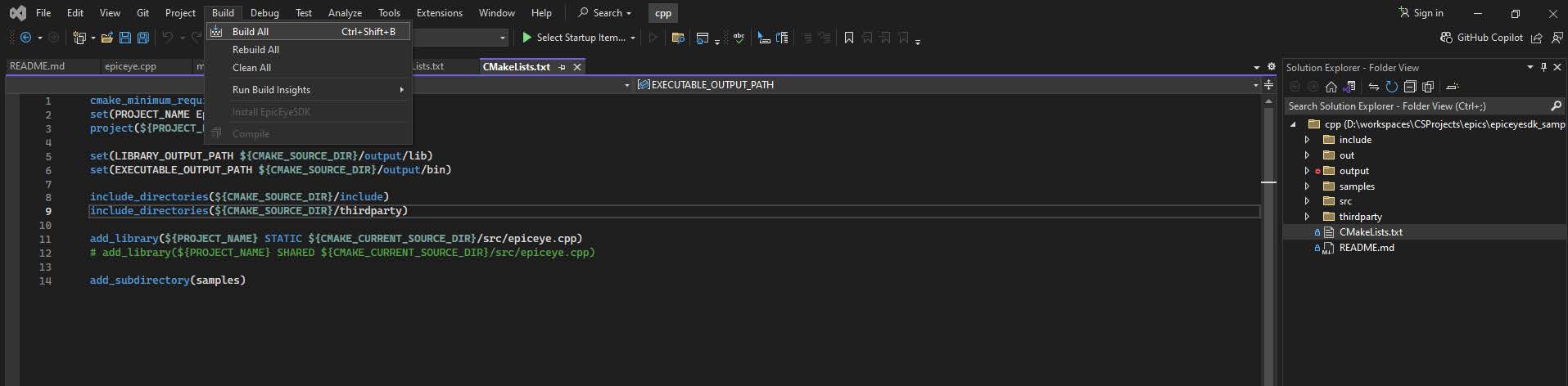
Select the desired object from the executor to run:
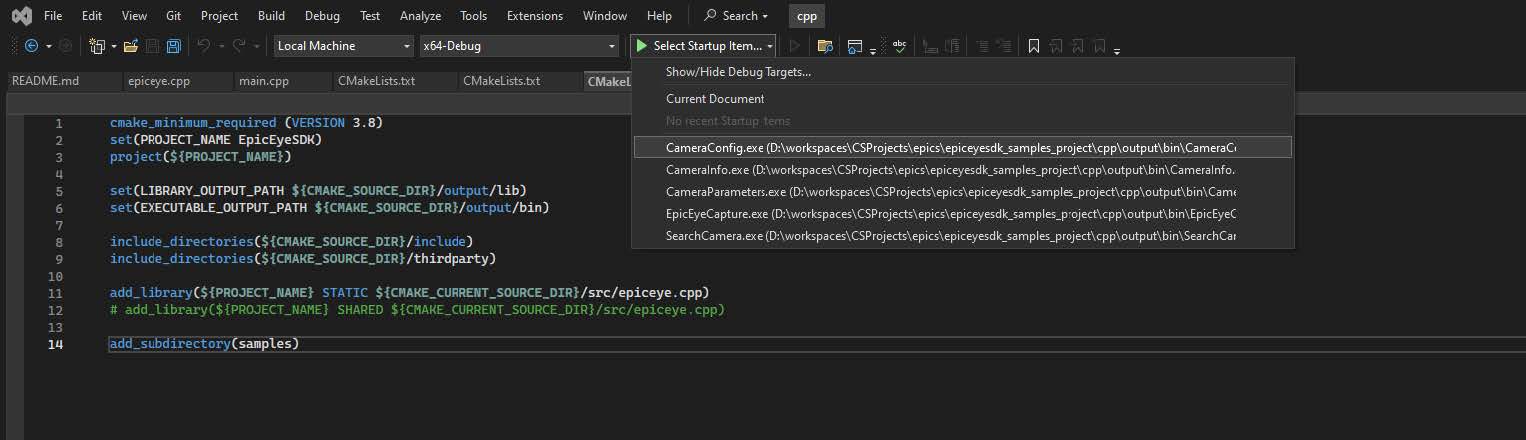
OpenCV Example
The sample project provides an EpicEyeCaptureOpencv program. Configure the OpenCV environment as follows:
-
Download the appropriate OpenCV version from Opencv release.
-
Extract to the project directory (epiceyesdk_samples/cpp/samples/EpicEyeCaptureOpencv/thirdparty).
-
Configure EpicEyeSDK in Visual Studio.
-
Click Build → Rebuild All to automatically copy OpenCV libraries to the binary output folder.
If you need to update the OpenCV version, modify the path in the ![]() |